The Python modulo operator (%) is a powerful tool that often gets overlooked by beginners. It’s a simple yet essential operator that helps you find the remainder when one number is divided by another.
Whether you’re working on loops, solving math problems, or managing odd and even numbers in your code, the modulo operator is a handy feature to master.
In this beginner-friendly guide, we’ll break down how the Python modulo operator works, explore its common uses, and provide easy examples to help you understand it better.
Also read: Understanding Python Sleep: How to python sleepPause Your Code with Time.sleep()
Understanding the % Operator: Unlocking the Power of Python Modulo
The % operator, also known as the modulo operator in Python, is a simple yet powerful tool that calculates the remainder when one number is divided by another.
It’s especially useful in programming for solving problems that require identifying patterns, performing conditional checks, or working with repetitive tasks like loops.
How Does the Modulo Operator Work?
The modulo operator follows this formula:
remainder = dividend % divisor
Here, the % administrator divides the dividend by the divisor and returns the remainder.
Example 1: Basic Usage
python
print(10 % 3) # Output: 1
print(15 % 4) # Output: 3
In these examples:
- 10 % 3 divides 10 by 3, resulting in a remainder of 1.
- 15 % 4 divides 15 by 4, leaving a remainder of 3.
Real-Life Use Cases of the Modulo Operator
Check for Even or Odd Numbers The % operator is a great way to determine whether a number is even or odd:
python
number = 7
if number % 2 == 0:
print(f”{number} is even.”)
else:
print(f”{number} is odd.”)
- Output: 7 is odd.
Cycle Through a Pattern Modulo can help you create repetitive patterns, such as alternating between two values:
python
for i in range(1, 6):
print(i % 2) # Output: 1, 0, 1, 0, 1
Work with Circular Indexing Imagine you have a list, and you want to cycle through it repeatedly:
python
items = [“apple”, “banana”, “cherry”]
for i in range(7):
print(items[i % len(items)])
Output:
apple
banana
cherry
apple
banana
cherry
apple
Why Learn the Modulo Operator?
The % operator may seem simple, but it’s a building block for many programming challenges. From creating dynamic patterns to solving algorithmic problems, understanding the modulo operator can enhance your Python skills and make your code cleaner and more efficient.
Practical Tips and Tricks for Mastering the Python Modulo Operator
Mastering the Python modulo operator (%) can help you write smarter, more efficient code. While the operator itself is simple, knowing how to use it effectively in different scenarios is key. Here are some practical tips and tricks to get the most out of the modulo operator.
1. Use Modulo for Cyclical Patterns
The modulo operator is ideally suited for making rehashing examples or burning through a grouping of significant worth. For instance, if you want to assign tasks to a group of three team members in a loop, the % operator can simplify your code:
python
team_members = [“Alice”, “Bob”, “Charlie”]
tasks = [“Task1”, “Task2”, “Task3”, “Task4”, “Task5”]
for i, task in enumerate(tasks):
print(f”{task} is relegated to {team_members[i % len(team_members)]}”)
Output:
vbnet
Task1 is assigned to Alice
Task2 is assigned to Bob
Task3 is assigned to Charlie
Task4 is assigned to Alice
Task5 is assigned to Bob
2. Check Divisibility Quickly
If you want to check whether a number is divisible by another (e.g., is 10 divisible by 5?), the modulo operator provides a quick solution:
python
number = 10
if number % 5 == 0:
print(f”{number} is divisible by 5.”)
else:
print(f”{number} is not divisible by 5.”)
Output: 10 is divisible by 5.
3. Avoid Division-Related Errors
When using the modulo operator, make sure the divisor is not zero. Dividing or applying modulo by zero will raise a ZeroDivisionError. Always validate inputs:
python
dividend = 15
divisor = 0
if divisor != 0:
print(dividend % divisor)
else:
print(“Error: Division by zero is not allowed.”)
4. Work with Negative Numbers
In Python, the % operator works seamlessly with negative numbers. The outcome generally takes the indication of the divisor:
python
print(-10 % 3) # Output: 2
print(10 % -3) # Output: -2
Understanding this behavior ensures that your calculations remain accurate, even when working with negative values.
5. Optimize Conditional Loops
The % operator is great for controlling actions inside loops, especially when you want something to happen every few iterations:
python
for i in range(1, 11):
if i % 3 == 0:
print(f”{i} is divisible by 3.”)
Output:
3 is divisible by 3.
6 is divisible by 3.
9 is divisible by 3.
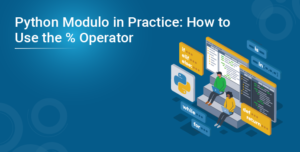
Advanced Applications of the Python Modulo Operator in Real-World Scenarios.
The Python modulo operator (%) is not just for basic tasks like finding remainders or checking even and odd numbers.
It also plays a vital role in solving advanced problems and implementing real-world solutions. Here are some examples of how the modulo operator is applied in practical scenarios to make coding smarter and more efficient.
1. Data Pagination
When building applications that display data in pages (like e-commerce sites or blogs), the modulo operator helps determine when to move to a new page. For example, if you’re displaying 10 items per page:
python
items = [“Product1”, “Product2”, “Product3”, “Product4”, “Product5”, “Product6”, “Product7”, “Product8”, “Product9”, “Product10”, “Product11”]
items_per_page = 10
for i, item in enumerate(items):
if i % items_per_page == 0 and i != 0:
print(“— New Page —“)
print(item)
Output:
python
Product1
Product2
…
Product10
— New Page —
Product11
2. Scheduling and Rotations
The modulo operator is excellent for scheduling tasks or creating rotation systems. For instance, if you have a set number of shifts or team members and want to rotate responsibilities:
python
team = [“Alice”, “Bob”, “Charlie”]
days = 7
for day in range(1, days + 1):
print(f”Day {day}: {team[(day – 1) % len(team)]} is on duty.”)
Output:
csharp
Day 1: Alice is on duty.
Day 2: Bob is on duty.
Day 3: Charlie is on duty.
Day 4: Alice is on duty.
…
3. Hashing and Data Bucketing
The modulo operator is often used in hash functions and database systems to distribute data into buckets. For example:
python
data = [15, 27, 36, 41, 59, 62]
buckets = 3
for num in data:
bucket_index = num % buckets
print(f”Data {num} goes into Bucket {bucket_index}”)
Output:
csharp
Data 15 goes into Bucket 0
Data 27 goes into Bucket 0
Data 36 goes into Bucket 0
Data 41 goes into Bucket 2
…
4. Clock Arithmetic
Modulo is fundamental for working with time and clock-based calculations. For example, converting a 24-hour format to a 12-hour clock:
python
hours_24 = 23
hours_12 = hours_24 % 12
print(f”12-hour format: {hours_12} PM”) # Output: 12-hour format: 11 PM
Optimizing Performance with the Python Modulo Operator in Large-Scale Applications
The Python modulo operator (%) is a powerful tool, but like any feature in programming, it can have performance implications when used in large-scale applications.
In scenarios involving huge datasets or intensive computation, optimizing how you use the modulo operator can help improve the efficiency of your code. Here are some practical tips for using % effectively to optimize performance in large-scale applications.
1. Avoid Redundant Calculations
In large-scale applications, especially those with repetitive operations, it’s crucial to avoid calculating the same modulo repeatedly. Caching results can save significant computational time.
For example, if you’re working with a large list of numbers and repeatedly checking if they are divisible by a fixed divisor, you can calculate the remainder once and store it:
python
divisor = 5
numbers = [10, 20, 30, 40, 50, 60]
mod_results = {}
# Cache the modulo results to avoid redundant calculations
for num in numbers:
if num not in mod_results:
mod_results[num] = num % divisor
print(mod_results)
By storing the modulo results, you avoid recalculating for each number multiple times in a loop, which is especially helpful when dealing with large data sets.
2. Efficient Handling of Large Datasets
In applications where you’re processing massive datasets, such as when analyzing logs, web traffic, or financial transactions, the modulo operator can help with things like data partitioning or load balancing. However, handling large datasets efficiently requires careful consideration. One strategy is to process data in chunks to reduce memory usage and speed up computation.
For example, instead of calculating the modulo for all records at once, process them in smaller batches:
python
def process_data_in_batches(data, batch_size):
for i in range(0, len(data), batch_size):
batch = data[i:i+batch_size]
for item in batch:
# Perform modulo operation here
print(item % 10)
# Example of large dataset
data = range(1, 1000000)
process_data_in_batches(data, 10000)
By breaking data into manageable chunks, you reduce the strain on memory and allow for more efficient processing of large datasets.
Conclusion
The Python modulo operator (%) may seem simple at first glance, but it’s a versatile tool that plays a crucial role in solving a wide range of programming challenges.
Whether you’re checking divisibility, working with cyclical patterns, or handling large datasets, understanding how to effectively use the modulo operator can greatly enhance your coding efficiency.
From basic applications like identifying even and odd numbers to advanced uses in data partitioning, scheduling, and performance optimization, the % operator proves to be indispensable in both small-scale scripts and large-scale systems.
By following the tips and techniques outlined in this guide, you can master the modulo operator, avoid common pitfalls, and use it in innovative ways to optimize your Python programs.